
Microservices are great, but not everything needs to be a microservice
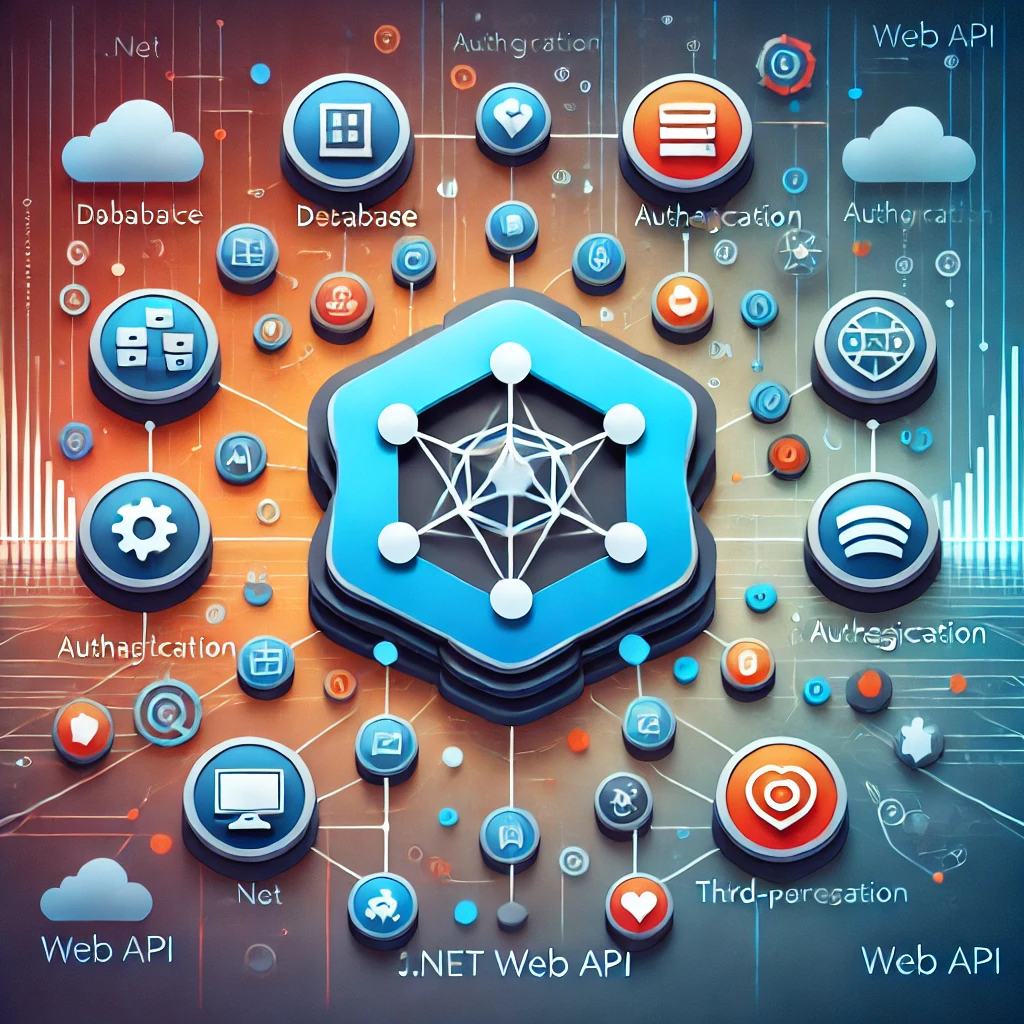
In today’s software development world, microservices have become a popular architectural style, offering a modular approach to building scalable and flexible applications. Microsoft Azure provides a powerful platform for hosting microservices, making it easier to deploy, manage, and monitor distributed systems. In this blog post, we’ll explore what microservices are with .NET C# examples, discuss the benefits and best practices of breaking down applications into microservices, and examine the drawbacks of overusing this architecture.
What is a Microservice?
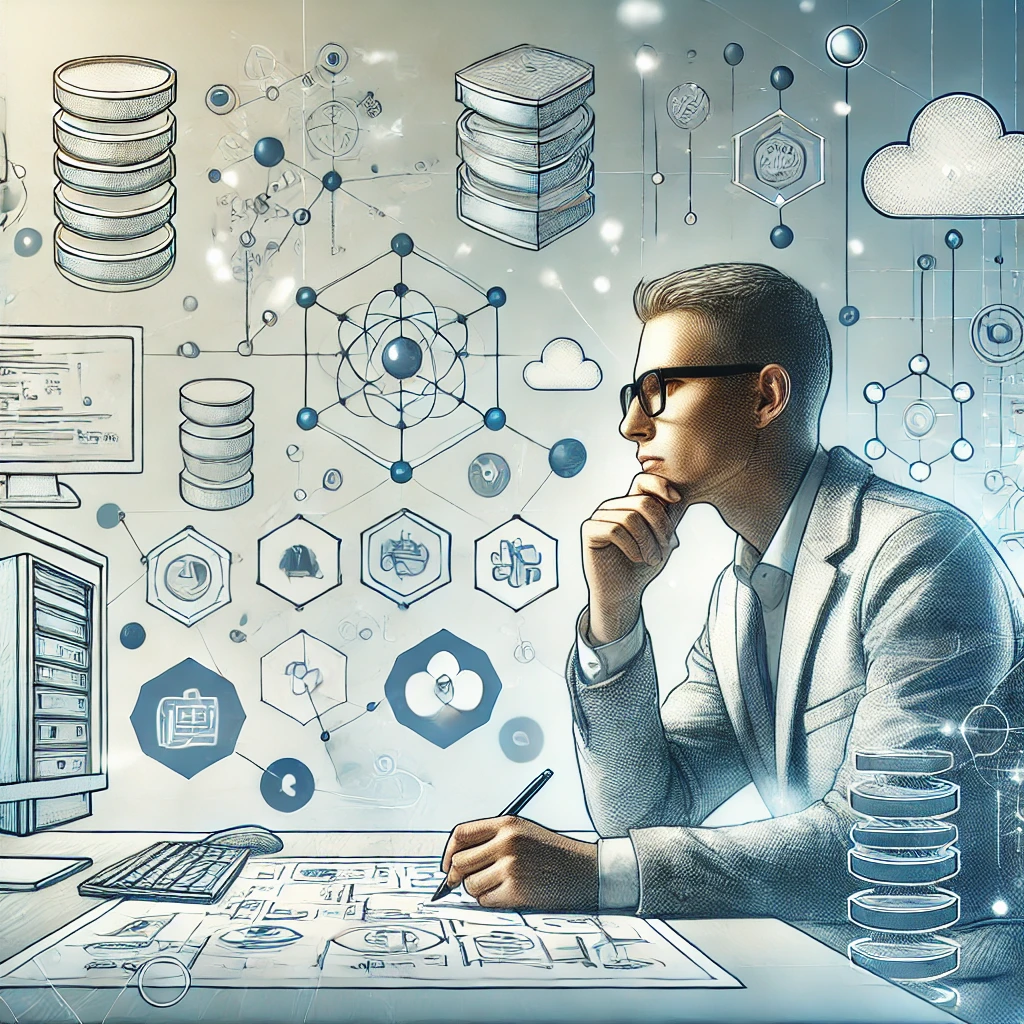
At its core, a microservice is a small, self-contained application designed to handle a specific business function. Unlike traditional monolithic architectures, where all functionalities are tightly coupled in a single codebase, microservices operate independently and communicate via APIs. This separation allows for more modular, scalable, and maintainable systems.
Example: A Simple Microservice in .NET C#
Let’s create a basic example of a microservice in .NET using ASP.NET Core. Imagine we’re building an e-commerce application, and we need a dedicated service to handle product inventory.
using Microsoft.AspNetCore.Mvc;
namespace InventoryService.Controllers
{
[ApiController]
[Route("api/[controller]")]
public class InventoryController : ControllerBase
{
private static readonly Dictionary<int, int> Inventory = new()
{
{ 1, 50 }, // Product ID 1 has 50 units in stock
{ 2, 30 }
};
[HttpGet("{productId}")]
public IActionResult GetStock(int productId)
{
if (Inventory.ContainsKey(productId))
{
return Ok(new { ProductId = productId, Stock = Inventory[productId] });
}
return NotFound(new { Message = "Product not found" });
}
[HttpPut("{productId}/{quantity}")]
public IActionResult UpdateStock(int productId, int quantity)
{
if (Inventory.ContainsKey(productId))
{
Inventory[productId] += quantity;
return Ok(new { ProductId = productId, Stock = Inventory[productId] });
}
return NotFound(new { Message = "Product not found" });
}
}
}
This service exposes RESTful endpoints to query and update product inventory. It can be deployed independently and scaled separately, ensuring that the inventory logic doesn’t interfere with other parts of the application.
Deploying the Microservice on Azure
To host this microservice on Azure, you can use Azure App Service for simple deployments or Azure Kubernetes Service (AKS) for more complex, containerized microservice environments.
1. Using Azure App Service:
- Publish the project to an Azure App Service using Visual Studio or Azure CLI.
- Enable Continuous Deployment for automated updates.
2. Using Azure Kubernetes Service (AKS):
Containerize the application using Docker:
FROM mcr.microsoft.com/dotnet/aspnet:6.0
COPY . /app
WORKDIR /app
ENTRYPOINT ["dotnet", "InventoryService.dll"]
Deploy the container to AKS using Helm or Kubernetes manifests.
Benefits and Best Practices for Breaking Down Applications into Microservices
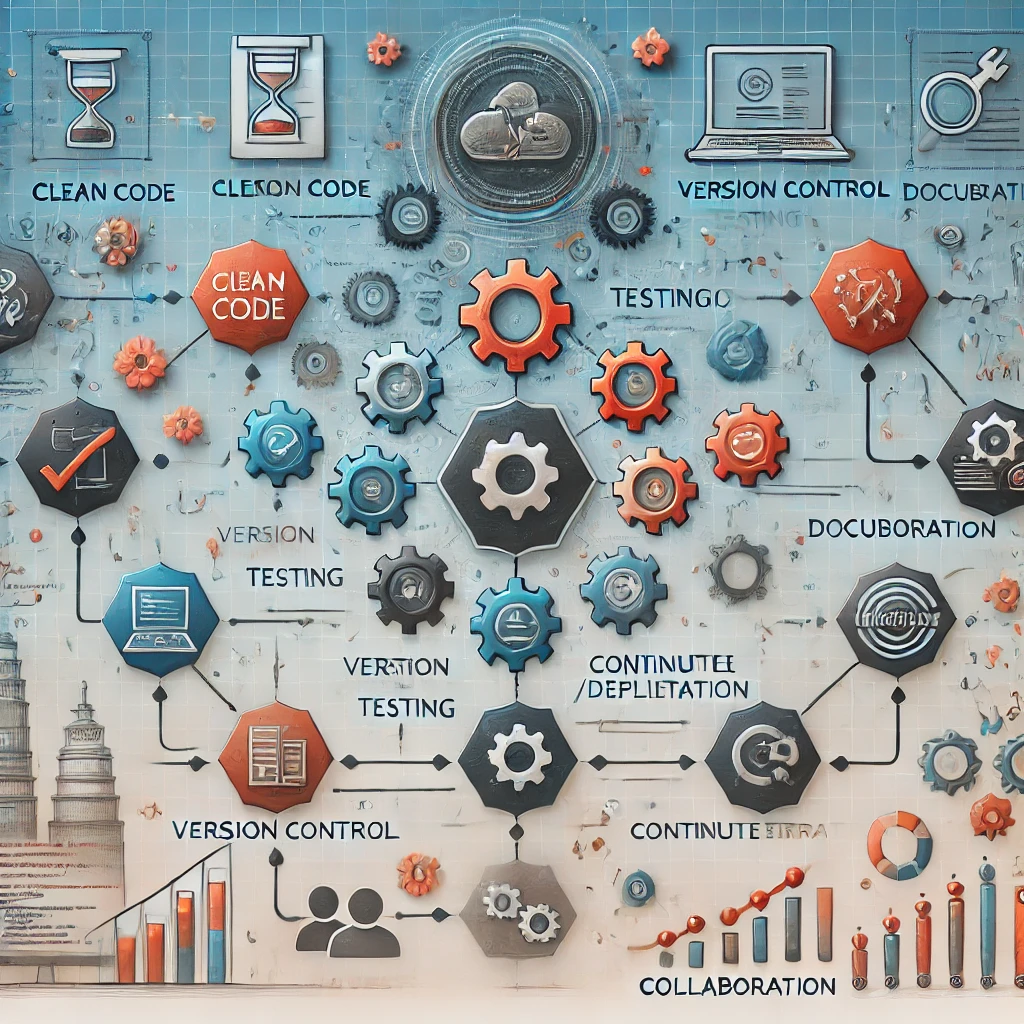
Microservices provide several advantages, but to reap their benefits, it’s essential to follow best practices. Here are key considerations:
Benefits of Microservices
- Scalability: Each microservice can be scaled independently based on its specific load, reducing resource usage.
- Technology Flexibility: Different teams can use different technologies best suited for their microservice.
- Faster Development Cycles: Teams can work on separate microservices simultaneously without impacting others.
- Fault Isolation: If one microservice fails, it doesn’t necessarily bring down the entire application.
- Easier Maintenance: Small, focused services are easier to update and debug than a monolithic application.
When and How to Break Down Applications
- Domain-Driven Design: Identify business domains and boundaries to create microservices that correspond to specific areas (e.g., user management, payments, inventory).
- High-Load Areas: Split components that experience high traffic or heavy computation (e.g., search functionality) into microservices.
- Frequent Changes: Isolate functionalities that change frequently, reducing the risk of breaking unrelated features.
- Team Ownership: Align microservices with team responsibilities to minimize cross-team dependencies.
The Drawbacks of Overusing Microservices
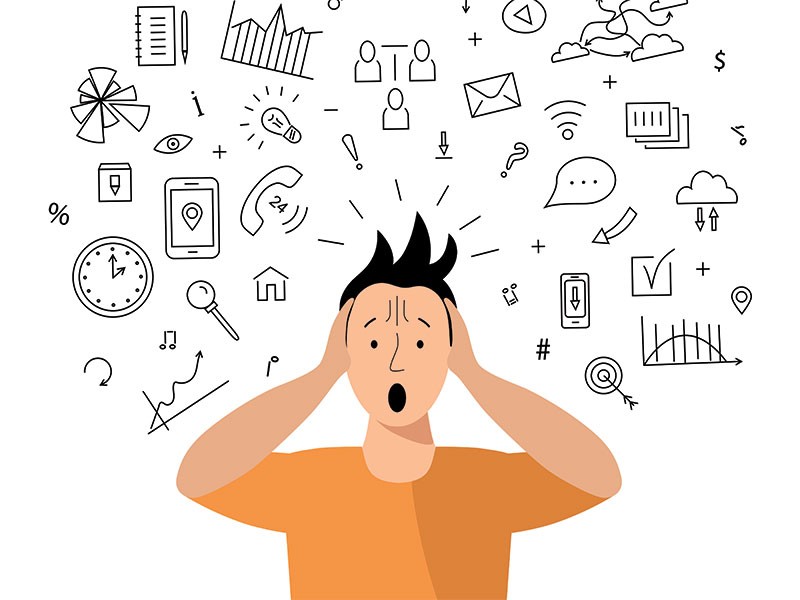
While microservices offer many benefits, overzealous adoption can lead to significant challenges. One of the most common pitfalls is trading simplicity in the codebase for complexity in infrastructure.
The Complexity Shift
In a monolithic architecture, the complexity is primarily contained within the codebase. However, in a microservices architecture, the complexity shifts to the infrastructure. Managing dozens or hundreds of services in Azure requires dealing with:
- Communication Overhead:
- Services need to communicate via APIs, often requiring load balancing, retries, and monitoring.
- Azure services like Azure API Management or Service Bus help manage communication but add layers of infrastructure.
- Deployment Challenges:
- Coordinating deployments of interdependent microservices can be tricky.
- Tools like Azure DevOps Pipelines or GitHub Actions are essential for managing CI/CD pipelines.
- Monitoring and Debugging:
- Observability becomes critical with distributed systems. Azure offers tools like Application Insights, Log Analytics, and Azure Monitor, but configuring them properly takes effort.
- Cost and Overhead:
- Small Applications: If the application is small and unlikely to grow significantly, a monolithic architecture may suffice.
- Tight Deadlines: Microservices introduce complexity that can slow down initial development.
- Inexperienced Teams: Without expertise in distributed systems and Azure infrastructure, microservices can become a maintenance nightmare.
Balancing Simplicity and Scalability
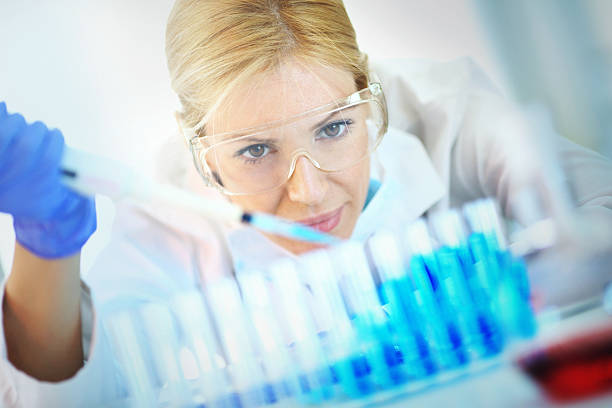
The key to a successful microservices architecture is balance. Start by identifying clear business needs for breaking down functionality. Avoid creating microservices just for the sake of it—every new service adds complexity to your Azure infrastructure.
Strategies for Managing Complexity
- Use Azure Service Mesh: Tools like Azure Service Fabric Mesh or Istio on AKS simplify communication and observability between microservices.
- Automate Infrastructure Management: Leverage Infrastructure as Code (IaC) tools like Azure Resource Manager (ARM) templates, Terraform, or Bicep.
- Centralize Monitoring: Use Azure Monitor to collect and analyze logs, metrics, and telemetry from all microservices.
- Optimize Costs: Continuously review Azure resource usage and eliminate unnecessary services or scale down idle resources.
Conclusion
Hosting microservices on Microsoft Azure unlocks incredible potential for scalability, flexibility, and maintainability. However, it’s essential to approach microservices with a clear strategy. By breaking down applications thoughtfully and managing infrastructure complexity, you can harness the benefits of microservices while avoiding common pitfalls.
While Azure has many great tools to help manage your infrastructure, it can be a real challenge to manage complexity within Azure infrastructure itself rather than in code. Ensuring that each microservice is created truly as it is needed rather than to “just create a microservice” is essential, and will help ensure you’re taking a thoughtful and meaningful approach to building out microservices.